1. What is ORM?
Keyword:Object-Relational Mapping.
converting data between relational databases and OOP languages.
Answer:
ORM is Object-Relational Mapping. It is a programming technique for converting data between incompatible type systems in relational databases and object-oriented programming languages. You can use an ORM framework to persist model objects to a relational database and retrieve them, and the ORM framework will take care of converting the data between the two otherwise incompatible states.
2. Explain first-level cache, second-level cache and query cache in Hibernate.
Keyword:First level cache: associated with session. enabled by default per transaction.
Second level cache: associated with session factory. disabled by default. Example: EHCache, OSCache, JBoss Cache.
Query cache: cache actual query results. disabled by default.
Answer:
First-level cache is associated with the Session object. By default, Hibernate uses first-level cache on a per-transaction basis. Hibernate uses this cache mainly to reduce the number of SQL queries it needs to generate within a given transaction. For example, if an object is modified several times within the same transaction, Hibernate will generate only one SQL UPDATE statement at the end of the transaction, containing all the modifications.
Second-level cache is associated with the Session Factory object. To reduce database traffic, second-level cache keeps loaded objects at the Session Factory level between transactions. These objects are available to the whole application, not just to the user running the query. This way, each time a query returns an object that is already loaded in the cache, one or more database transactions potentially are avoided. EHCache, OSCache, JBoss Cache are examples of second-level cache provider.
By default, second-level cache is not enabled, you need to configure it as follows:
<property key="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">net.sf.ehcache.hibernate.EhCacheRegionFactory</property>
Query cache is used to cache actual query results, rather than just persistent objects. Query cache should always be used in conjunction with the second-level cache.
By default, query cache is not enabled. To enable query cache, the following property should be used:
<property key="hibernate.cache.use_query_cache">true</property>
3. What is lazy loading in Hibernate?
Keyword:parent-children, doesn’t load the children when loading the parent,
lazy=true/false,
parent.getChildren().size()
Answer:
In a one-to-many relationship, lazy setting decides whether to load child objects while loading the Parent Object.
lazy=true means Hibernate doesn’t load the children when loading the parent. This is the default behavior.
lazy=false makes Hibernate load the children when parent is loaded from the database.
Example:
public class Parent {
private Set<Child> children;
public Set<Child> getChildren() {
return children;
}
}
public void process() {
//children contains nothing because of lazy loading.
Set<Child> children = parent.getChildren();
// When call one of the following methods,
// Hibernate will start to actually load and fill the set.
children.size();
children.iterator();
}
4. What are the different Cascade: DELETE and DELETE-ORPHAN?
Keyword:DELETE: delete referenced children when parent entity is deleted.
DELETE-ORPHAN: delete referenced children marked as removed (orphans) when parent entity is saved or updated.
Answer:
Cascade DELETE means if one parent entity is deleted, its referenced children will be deleted automatically.
Example:
Query query = session.create("from Parent where id = :id");
query.setParameter("id", 123);
Parent parent = (Parent)query.list().get(0);
session.delete(parent); //parent’s children will be deleted as well
Cascade DELETE-ORPHAN means when save or update one parent entity, only those children that have been marked removed will be deleted automatically. DELETE-ORPHAN allows parent table to delete few records (orphans) in child table.
Example:
Child c1 = (Child)session.get(Child.class, new Integer(10));
Child c2 = (Child)session.get(Child.class, new Integer(20));
Set<Child> children = parent.getChildren();
children.remove(c1); //c1 mark as removed
children.remove(c2); //c2 mark as removed
session.saveOrUpdate(parent); //c1 and c2 will be deleted from table as well.
5. What is the difference between JPA and Hibernate?
Answer:JPA is a specification for implementing ORM. It provides a set of guidelines that JPA implementation vendors should follow to create an ORM implementation.
Hibernate is an popular provider of JPA specification.
6. How to define a JPA entity class?
Answer:The following is an example to define a typical JPA entity class
@Entity //annotate entity class
@Table(name = "user") //table mapping
public class User implements Serializable {
//property declaration
private Integer id;
private String firstName;
public User() {
//default constructor
}
//primary key
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "user_id")
public Integer getId() {
return this.id;
}
public void setId(Integer id) {
this.id = id;
}
//column mapping
@Column(name = "first_name")
public String getFirstName() {
return this.firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
}
7. Describe JPA Annotations for relationship definition.
Keyword:@OneToOne, @OneToMany, @ManyToOne, @ManyToMany
Answer:
JPA use the following annotations to specify the relationships between entity classes:
@OneToOne defines a single-valued association to another entity that has one-to-one multiplicity.
Example:
// On Customer class:
@OneToOne
@JoinColumn(name="custom_information_id")
public CustomerInformation getCustomerInformation() { return customerInformation; }
// On CustomerInformation class:
@OneToOne(mappedBy="customerInformation")
public Customer getCustomer() { return customer; }
@OneToMany defines a many-valued association with one-to-many multiplicity.
Example:
// In Customer class:
@OneToMany(cascade=ALL, mappedBy="customer")
public Set<Order> getOrders() { return orders; }
// In Order class:
@ManyToOne
@JoinColumn(name="customer_id", nullable=false)
public Customer getCustomer() { return customer; }
@ManyToOne defines a single-valued association to another entity class that has many-to-one multiplicity.
Example:
@ManyToOne(optional=false)
@JoinColumn(name="customer_id", nullable=false, updatable=false)
public Customer getCustomer() { return customer; }
@ManyToMany defines a many-valued association with many-to-many multiplicity.
Example:
// In Customer class:
@ManyToMany
@JoinTable(name="customer_product")
public Set<Product> getProducts() { return products; }
// In Product class:
@ManyToMany(mappedBy="products")
public Set<Customer> getCustomers() { return customers; }
8. What is the difference between @JoinColumn and mappedBy attribute?
Answer:@JoinColumn annotation indicates that this entity is the owner of the relationship, the corresponding table has a column with a foreign key to the referenced table.
mappedBy attribute indicates that the entity in this side is the inverse of the relationship, and the owner resides in the "other" entity.
An example:
@Entity
public class Company {
@OneToMany(fetch = FetchType.LAZY, mappedBy = "company")
private Set<Department> departments;
}
@Entity
public class Department {
@ManyToOne
@JoinColumn(name = "companyId")
private Company company;
}
9. What is @Temporal in JPA?
Answer:@Temporal annotation is used to convert the date and time values between Java object and compatible database type. @Temporal must be specified for persistent fields or properties of type java.util.Date and java.util.Calendar. It may only be specified for fields or properties of these types.
@Temporal has three type of values:
TemporalType.DATE
TemporalType.TIME
TemporalType.TIMESTAMP
An example:
@Temporal(TemporalType.DATE)
@Column(name = "register_date")
private java.util.Date registerDate;
10. What is the difference between JPQL and Criteria API?
Answer:JPQL queries are defined as strings, similarly to SQL. JPA criteria queries, on the other hand, are defined by instantiation of Java objects that represent query elements.
A major advantage of using the criteria API is that errors can be detected earlier, during compilation rather than at runtime. On the other hand, for many developers string based JPQL queries, which are very similar to SQL queries, are easier to use and understand.
For simple static queries - string based JPQL queries may be preferred. For dynamic queries that are built at runtime - the criteria API may be preferred.
More Java JPA & Hibernate interview questions and answers: Java Interview Notes
- What is SessionFactory in Hibernate?
- Explain Hibernate object states.
- What is the difference between merge method and update method in Hibernate?
- What is Transaction in Hibernate?
- What are the advantages of using JPA?
- What is Entity in JPA?
- What is Persistence Unit?
- What is Entity Manager
- What is FetchType in JPA?
- What is the difference between JPQL and HQL?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.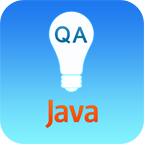
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play