1. Explain the multi-tiered architecture in Java EE.
Keyword:Web tier: Servlet, JSP, JSF, Spring MVC, Struts,
Business tier: Spring IOC, EJB, REST, Web services,
Data tier: JDBC, JPA, Hibernate.
Answer:
In a multi-tiered application, the functionality is separated into isolated functional areas, called tiers. In a typical Java EE architecture, there are the following tiers:
The client tier
The client tier consists of application clients that access a Java EE server and that are usually located on a different machine from the server. Clients can be a web browser, a standalone application, or other servers, and they run on a different machine from the Java EE server.
The Web Tier
The web tier consists of components that handle the interaction between clients and the business tier.
Its primary tasks are:
Dynamically generate content in various formats for the client.
Collect input from users of the client interface and return appropriate results from the components in the business tier.
Control the flow of screens or pages on the client.
Maintain the state of data for a user's session.
Perform some basic logic and hold some data temporarily in JavaBeans components.
Technologies used in the Web Tier:
Servlets, JSP, JSF, JSTL, EL, JavaBeans, Spring MVC, Struts, etc.
The Business Tier
The business tier consists of components that provide the business logic for an application. In a properly designed enterprise application, the core functionality exists in the business tier components.
Technologies Used in the Business Tier:
EJB, Spring IOC, POJO, JAX-RS RESTful web services, JAX-WS web services.
The Data Tier or EIS Tier
The data tier (often called the enterprise information systems tier, or EIS tier) consists of database servers, enterprise resource planning systems, and other legacy data sources, like mainframes. These resources typically are located on a separate machine than the Java EE server, and are accessed by components on the business tier.
Technologies Used in the Data Tier:
JDBC, JPA, Hibernate, Java EE Connector Architecture (JCA), Java Transaction API (JTA).
2. Explain the life cycle of Servlet.
Keyword:Servlet container load servlet class,
init() create servlet instance,
service(), doGet(), doPost() handle request,
destroy() remove servlet.
Answer:
The life cycle of a servlet is controlled by the servlet container. When a request is mapped to a servlet, the container performs the following steps:
If an instance of the servlet does not exist, the servlet container loads the servlet class;
The servlet container creates an instance of the servlet class by calling the servlet’s init() method;
The servlet container invokes the service method of the servlet, passing request and response objects. The service() method checks the HTTP requests type (GET, POST) and calls the doGet() or doPost method;
If the container needs to remove the servlet, it finalizes the servlet by calling the servlet’s destroy() method.
3. What is the difference between ServletConfig and ServletContext?
Keyword:ServletConfig, servlet level, access within servlet.
ServletContext, web application level, available for all servlet within the application.
web.xml: <init-param>, <context-param>.
Answer:
ServletConfig is specific to a particular servlet. It is used to pass information to a servlet during initialization. The parameters configured in a ServletConfig object can only be accessed within the servlet.
ServletContext defines a set of methods that a servlet uses to communicate with its servlet container. There is one ServletContext instance per web application per JVM. The parameters configured at the ServletContext level are available to all servlets within the application.
In web.xml, you can define ServletContext parameters under <web-app> tag, you can define ServletConfig parameters under <servlet> tag. For example:
<web-app>
<context-param>
<param-name>servlectContextParam1</param-name>
<param-value>servlectContextValue1</param-value>
</context-param>
......
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.xxx.MyServlet</servlet-class>
<init-param>
<param-name>myServletConfigParam1</param-name>
<param-value>myServletConfigValue1</param-value>
</init-param>
</servlet>
......
</web-app>
4. Are Servlets thread Safe? How to make a servlet thread safe?
Keyword:Only one instance for every servlet.
Avoid access/reassign member variable,
Avoid synchronize the service (doGet, doPost) methods,
Implement SingleThreadModel interface, deprecated.
Answer:
No, servlets are not thread safe. In servlet container, there is only one instance for every servlet. Servlet container may send concurrent requests through the service method of the servlet.
To make sure that a servlet is thread safe:
a. The service method of servlet should not access any member variables, unless these member variables are thread safe themselves.
b. The service method of servlet should not reassign member variables, as this may affect other threads executing inside the service() method. If it is really necessary to reassign a member variable, make sure this is done inside a synchronized block.
c. Avoid to synchronize the service (or doPost, doGet) method directly, use synchronized block instead.
d. Implement SingleThreadModel interface to create a single-threaded servlet. But SingleThreadModel interface is deprecated, it is not recommended to use it.
5. What is Java EE Container?
Answer:Java EE containers are the interface between Java EE components and the lower-level functionality provided by Java EE platform. The functionality of the container is defined by the platform, and is different for each component type. Java EE server allows the different component types to work together to provide functionality in an enterprise application.
Java EE Containers provide configurable services such as security, transaction management, JNDI lookups, and remote connectivity. Java EE containers also manage nonconfigurable services, such as enterprise bean and servlet lifecycles, database connection resource pooling, data persistence, and access to the Java EE platform APIs.
6. What is Java EE Component?
Answer:A Java EE component is a self-contained functional software unit that is assembled into a Java EE application with its related classes and files and that communicates with other components. Java EE applications are made up of components.
The Java EE specification defines the following Java EE components:
Application clients and applets are components that run on the client.
Java Servlet, JavaServer Faces, and JavaServer Pages (JSP) technology components are web components that run on the server.
EJB components (enterprise beans) are business components that run on the server.
7. Explain the basic steps of using JDBC.
Answer:Basically a Java program implementing JDBC performs the following steps:
1). Load a JDBC driver.
2). Establish a database connection through a connection URL.
3). Create a statement object.
4). Execute a query or update on the statement object.
5). Process the result.
6). Close the connection.
Example:
Connection conn = null;
Statement stmt = null;
try{
//STEP 1:
Class.forName("com.mysql.jdbc.Driver");
//STEP 2:
conn = DriverManager.getConnection("jdbc:mysql://hostname:port/mydb","user", "pwd")
//STEP 3:
stmt = conn.createStatement();
//STEP 4:
String sql = "SELECT id, name FROM Users";
ResultSet rs = stmt.executeQuery(sql);
//STEP 5:
while(rs.next()){
int id = rs.getInt("id");
......
}
//STEP 6:
rs.close();
stmt.close();
conn.close();
}catch(SQLException e1){
......
}catch(Exception e2){
......
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException e3){}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){}
}
8. What is the difference between Point-to-Point Messaging and Publish/Subscribe Messaging?
Keyword:Point-to-Point Messaging: queue, sender, receiver.
Publish/Subscribe Messaging: topic, publisher, subscriber.
Answer:
A point-to-point (PTP) application is built on the concept of message queues, senders, and receivers. Each message is addressed to a specific queue, and receiving clients extract messages from the queues established to hold their messages. Queues retain all messages sent to them until the messages are consumed or expire.
PTP messaging has the following characteristics:
a. Each message has only one consumer.
b. A sender and a receiver of a message have no timing dependencies. The receiver can fetch the message whether or not it was running when the client sent the message.
c. The receiver acknowledges the successful processing of a message.
In a publish/subscribe (pub/sub) application, clients address messages to a topic. Publishers and subscribers can dynamically publish or subscribe to the content hierarchy. The system takes care of distributing the messages arriving from a topic’s multiple publishers to its multiple subscribers. Topics retain messages only as long as it takes to distribute them to current subscribers.
Pub/sub messaging has the following characteristics.
Each message can have multiple consumers.
Publishers and subscribers have a timing dependency. A client that subscribes to a topic can consume only messages published after the client has created a subscription, and the subscriber must continue to be active in order for it to consume messages.
9. What are RESTful Web Services?
Keyword:REST, resources, URI.
JAX-RS
Answer:
RESTful web services are loosely coupled, lightweight web services that are particularly well suited for creating APIs for clients spread out across the internet.
Representational State Transfer (REST) is an architectural style of client-server application centered around the transfer of representations of resources through requests and responses.
In the REST architectural style, data and functionality are considered resources and are accessed using Uniform Resource Identifiers (URIs), typically links on the Web. The resources are represented by documents and are acted upon by using a set of simple, well-defined operations.
In Java EE, we can use Java API for RESTful Web Services (JAX-RS) to build RESTful web services.
10. What are the differences between SOAP-based Web Services and RESTful Web Services?
Answer:SOAP-based web services is a heavyweight solution. It has the following advantages:
a. Language, platform, and transport independent (HTTP or SMTP), while REST is based on HTTP;
b. Works well in distributed enterprise environments, while REST assumes direct point-to-point communication;
c. Reliable. SOAP provides reliable messaging (WS-ReliableMessaging) and end to end reliability through soap intermediaries.
d. Security. support different standards for security. WS-Security provides end-to-end security covering message integrity and authentication.
REST is a lightweight solution. It is easier to use and is more flexible. It has the following advantages:
a. No expensive tools require to interact with the Web service;
b. Smaller learning curve;
c. Flexible data representation: SOAP uses XML for all messages, REST can use XML, JSON or other format;
d. Performance: RESTful Web services are completely stateless. Restful services provide a good caching infrastructure over HTTP GET method.
More Java EE interview questions and answers: Java Interview Notes
- What is the difference between Java EE and Java SE?
- Explain MVC architecture relating to Java EE.
- What is the difference between doGet and doPost method?
- What is the difference between sendRedirect and forward methods?
- What is the difference between Java EE component and standard Java class?
- What is the difference between Statement, PreparedStatement and CallableStatement?
- What is the difference between Topic and Queue?
- What is CDI in Java EE?
- Explain the main principles of RESTful Web Services.
- What is JAX-RS API?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.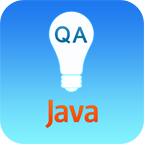
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
Informative post, thanks for sharing.
ReplyDeleteSpring Training in Chennai | Hibernate Training in Chennai | Struts Training in Chennai
Informative post indeed, I’ve being in and out reading posts regularly and I see alot of engaging people sharing things and majority of the shared information is very valuable and so, here’s my fine read.
ReplyDeleteclick here to confirm
click here download
click here digital
click here download cb background
click here download the videos
Nice article. It is unique and fantastic information.
ReplyDeleteAngularJS training in chennai | AngularJS training in anna nagar | AngularJS training in omr | AngularJS training in porur | AngularJS training in tambaram | AngularJS training in velachery
MMORPG OYUNLARI
ReplyDeleteİnstagram Takipçi Satin Al
tiktok jeton hilesi
TİKTOK JETON HİLESİ
SAÇ EKİM ANTALYA
İnstagram Takipçi Satın Al
ınstagram takipci satin al
metin2 pvp serverlar
instagram takipçi satın al
smm panel
ReplyDeleteSmm panel
İS İLANLARİ BLOG
İNSTAGRAM TAKİPÇİ SATIN AL
HIRDAVAT
BEYAZESYATEKNİKSERVİSİ.COM.TR
SERVİS
tiktok jeton hilesi
pendik samsung klima servisi
ReplyDeleteçekmeköy samsung klima servisi
ataşehir samsung klima servisi
pendik mitsubishi klima servisi
tuzla vestel klima servisi
tuzla bosch klima servisi
tuzla arçelik klima servisi
kartal toshiba klima servisi
beykoz daikin klima servisi