1. What is Spring Framework? What are the advantages of using Spring?
Keyword:application framework, IOC container.
Non-invasive development with POJO,
Loose coupling through dependency injection and interface orientation,
Spring AOP provides declarative common services, security, transaction, logging,
Reduce boilerplate code through aspects and templates. JdbcTemplate.
Answer:
The Spring Framework is an open source application framework and inversion of control (IOC) container for developing Java applications. Spring enables you to build applications from "plain old Java objects" (POJOs) and to apply enterprise services non-invasively to POJOs.
The main advantages of using Spring Framework are:
a. Lightweight and minimally invasive development with Plain Old Java Objects (POJOs). A Spring component can be any type of POJO. You don't need to implemented Spring specific interfaces or extend Spring specific classes.
b. Loose coupling through dependency injection and interface orientation. Spring IoC container manages java objects – from instantiation to destruction – through its BeanFactory, leaving them loosely coupled and allowing you to code to abstractions and to write testable code. For example, injecting mock implementation during testing.
c. Spring AOP provides declarative common services, like security, transaction, logging, leaving the application components to focus on their specific business logic;
d. Boilerplate reduction through aspects and templates. For example, Spring JdbcTemplate encapsulates JDBC checked exceptions to meaningful runtime exceptions.
2. What is IOC?
Keyword:Inversion of Control, Dependency Injection.
Object coupling is bound at run time by an assembler object.
Answer:
Inversion of Control (IOC) is an object-oriented programming technique, in which object coupling is bound at run time by an assembler object and is typically not known at compile time using static analysis. IOC is also known as Dependency Injection (DI). In traditional programming, the flow of the business logic is determined by objects that are statically assigned to one another. With inversion of control, the flow depends on the object graph that is instantiated by the assembler and is made possible by object interactions being defined through abstractions. The binding process is achieved through dependency injection.
Example code:
Service: UserService interface and its implementations DbUserService, MockUserService
Client: UserManager
//User Service interface with a checkUser method
public interface UserService {
boolean checkUser(String userName, String password);
}
//User Service implementation: check user via database
public class DbUserService implements UserService{
public boolean checkUser(String userName, String password);
//connect to database and check user
}
}
//User Service mock implementation: check user via test data
public class MockUserService implements UserService{
//define and init test user data.
private Map<String, String> testUsers = ......;
public boolean checkUser(String userName, String password);
//check user using test data
if(testUsers.get(userName)) {
......
}
}
}
//Traditional implementation without IOC:
//UserManager and UserService are tightly coupled,
//Cannot change another implementation at runtime, hard to test.
public class UserManager {
private UserService userService;
public UserManager() {
this.userService = new DbUserService();
}
}
//Implementation with IOC:
//UserManager and UserService are loosely coupled,
//The dependency is injected at runtime, can be either DbUserService or MockUserService,
//It is easy to test by injecting a mock implementation.
public class UserManager {
private UserService userService;
public UserManager(UserService userService) {
this.userService = userService;
}
}
3. What is Spring Container?
Keyword:IOC container, manage components lifecycle.
Bean Factory, Application Context.
Answer:
Spring Container is at the core of the Spring Framework. It uses dependency injection (DI) to manage the components. In a Spring-based application, your application objects will live within the Spring container. The container will create the objects, wire them together, configure them, and manage their complete lifecycle from cradle to grave.
There are two types of Spring Container:
a. Bean factories (defined by the org.springframework.beans.factory.BeanFactory interface) are the simplest of
containers, providing basic support for DI.
b. Application contexts (defined by the org.springframework.context.ApplicationContext interface) build on the notion
of a bean factory by providing application framework services, such as the ability to resolve textual messages from a properties file and the ability to publish application events to interested event listeners.
4. Explain Spring Bean lifecycle.
Answer:a. Spring instantiates the bean.
b. Spring injects values and bean references into the bean’s properties.
c. If the bean implements BeanNameAware, Spring passes the bean’s ID to the setBeanName() method.
d. If the bean implements BeanFactoryAware, Spring calls the setBeanFactory() method.
e. If the bean implements ApplicationContextAware, Spring will call the setApplicationContext() method.
f. If the bean implements the BeanPostProcessor interface, Spring calls the postProcessBeforeInitialization() method.
g. If the bean implements the InitializingBean interface, Spring calls the afterPropertiesSet() method. Similarly, if the bean was declared with an init-method, then the specified initialization method will be called.
h. If the bean implements BeanPostProcessor, Spring will call the postProcessAfterInitialization() method.
i. At this point, the bean is ready to be used by the application and will remain in the application context until the application context is destroyed.
j. When container is shut down, if the bean implements the DisposableBean interface, then Spring will call the destroy() method. Likewise, if the bean was declared with a destroy-method, then the specified method will be called.
5. How to customize bean init and destroy process?
Keyword:init-method, destroy-method.
default-init-method, default-destroy-method.
Implement InitializingBean and DisposableBean interfaces.
Answer:
There are several ways to customize bean init and destroy process:
a. Declare the <bean> with init-method and destroy-method parameters. For example:
public class MyBean {
public void doInit() { //customized init code}
public void doDestroy() { //customized destroy code}
}
<bean id="mybean" class="MyBean" init-method="doInit" destroy-method="doDestroy"/>
b. Set default-init-method and default-destroy-method in context definition file:
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"
default-init-method="doInit"
default-destroy-method="doDestroy"> ...
</beans>
This setting will apply to all beans. You don’t have to declare init-method or destroy-method on each individual bean.
c. Implement InitializingBean and DisposableBean interfaces.
InitializingBean declares an afterPropertiesSet() method that serves as the init method.
DisposableBean declares a destroy() method that gets called when a bean is removed from the application context.
6. What is the difference between <context:annotation-config> and <context:component-scan>?
Answer:<context:annotation-config> is used to activate annotations in beans already registered in the application context (no matter if they were defined with XML or by package scanning).
<context:component-scan> can also do what <context:annotation-config> does but also scans packages to detect and register Spring stereotyped classes within the ApplicationContext, for example: @Controller, @Service, @Repository, @Component.
7. Explain Spring stereotype annotations: @Component, @Service, @Repository, @Controller.
Answer:@Component is a generic stereotype for any Spring-managed component. Classes marked with @Component are considered as candidates for auto-detection when using annotation-based configuration and classpath scanning.
@Repository, @Service, and @Controller are special kinds of @Component:
@Repository annotate DAO classes.
@Service annotate business logic (or called Business Service Facade) classes.
@Controller annotate Spring web controllers.
8. Describe the workflow of Spring Web MVC.
Keyword:DispatcherServlet -> handler mapping -> controller -> ModelAndView -> ViewResolver -> view
Answer:
a. The DispatcherServlet configured in web.xml file receives the request.
b. DispatcherServlet consults one or more handler mappings to figure out the controller that is responsible for processing the request.
c. DispatcherServlet sends the request to the chosen controller. The controller handles the request by invoking service objects and business logic.
d. Controller packages up the model data as a map and identify the name of a view that should render the output. It sends the request, model and view name (a ModelAndView instance) back to the DispatcherServlet.
e. DispatcherServlet consults the ViewResolver to map the view name to a specific view implementation.
f. DispatcherServlet sends the model data to the view implementation. The view uses the model data to render the output.
g. DispatcherServlet sends the output to servlet container and finally servlet sends the response back to the user.
9. Can we configure a Spring Web MVC application without using web.xml?
Keyword:Servlet 3.0, WebApplicationInitializer
Answer:
Yes. In a Servlet 3.0+ environment, we can configure the Servlet container programmatically as an alternative or in combination with a web.xml file. To do it, we just need to implement WebApplicationInitializer interface. WebApplicationInitializer is an interface provided by Spring MVC that ensures your implementation is detected and automatically used to initialize any Servlet 3 container.
For example:
public class SampleApplicationInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext container) {
XmlWebApplicationContext appContext = new XmlWebApplicationContext();
appContext.setConfigLocation("/WEB-INF/spring/iraylab-sample.xml");
ServletRegistration.Dynamic registration = container.addServlet("iraylab-sample", new DispatcherServlet(appContext));
registration.setLoadOnStartup(1);
registration.addMapping("/");
}
}
10. How to handle static resources in Spring Web MVC?
Answer:Use <mvc:resources> element in context definition file.
<mvc:resources> sets up a handler for serving static content.
<beans xmlns="http://www.springframework.org/schema/beans" ......>
<mvc:resources mapping="/resources/**" location="/resources/"/>
</beans>
More Java Spring Framework interview questions and answers: Java Interview Notes
- What are the different types of IOC (dependency injection)?
- What is the difference between BeanFactory and ApplicationContext?
- How to configure a singleton class as a bean in Spring container?
- How to configure lazy-initialized bean in Spring?
- How to configure bean automatic detection?
- Explain AOP concepts: Aspect, Joint Point, Advice, Pointcut, Weaving.
- Explain DAO support in Spring.
- What is @Controller in Spring Web MVC?
- What is @RequestMapping in Spring Web MVC?
- How to validate @Controller inputs in Spring Web MVC?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.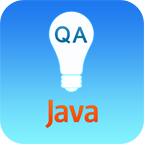
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
No comments:
Post a Comment