1. Explain jQuery main features.
Answer:jQuery includes the following features:
DOM element selections, traversal and modification;
DOM manipulation based on CSS selectors;
HTML Event methods;
Effects and animations;
AJAX;
Extensibility through plug-ins;
Utilities - such as user agent information, feature detection;
Multi-browser support.
2. How to include jQuery in your web pages?
Keyword:<script> tag
CDN
Answer:
There are two ways to include jQuery:
a. Download jQuery library and add the jQuery file in HTML <script> tag in <head> section.
For example:
<!DOCTYPE html>
<html>
<head>
<script src="jquery-{version}.min.js"></script>
</head>
b. Include jQuery from a CDN.
For example, use jQuery from Google CDN:
<!DOCTYPE html>
<html>
<head>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/{version}/jquery.min.js">></script>
</head>
3. What is the different between window.onload and $(document).ready?
Keyword:window.onload: after all content (css, images) of web page loaded,
$(document).ready: after DOM loaded, before css, images loaded.
Answer:
window.onload is a built-in Javascript event that occurs when all content of the web page has been loaded, including css, images, etc.
$(document).ready is jQuery event that occurs as soon as the HTML DOM is loaded, before css, images and other resources are loaded.
We can add multiple document.ready() function in a page, while we can add only one window.onload function.
4. What is jQuery.noConflict?
Keyword:relinquish jQuery's control of the $ variable
Answer:
jQuery.noConflict is used to relinquish jQuery's control of the $ variable.
Many JavaScript libraries use $ as a function or variable name, just as jQuery does. If you need to use another JavaScript library alongside jQuery, return control of $ back to the other library with a call to $.noConflict().
Example:
<script src="other_lib.js"></script>
<script src="jquery.js"></script>
<script>
$.noConflict();
// Code that uses other library's $ can follow here.
//......
//Use jQuery for jQuery code
jQuery(document).ready(function(){
jQuery( "div p" ).hide();
});
</script>
5. Explain different selectors in jQuery.
Keyword:element selector, id selector, class selector, attribute selector, etc
Answer:
jQuery offers a powerful set of selectors for selecting and manipulating HTML element(s).
a. All Selector ("*")
$("*") Selects all elements
b. Element Selector ("element")
Selects all elements with the given tag name.
Example:
$("p") Select all <p> elements on a page
c. ID Selector ("#id")
Selects a single element with the given id attribute.
Example:
$("#book") Select the element with id="book"
d. Class Selector (".class")
Selects all elements with the given class.
Example:
$(".author") Select all elements with class="author"
e. Attribute Selector
Selects elements that have the specified attribute with a value matches a certain matching rule.
Example:
$( "input[value='Submit']" ) Select all input elements that "value" attribute equals "Submit"
$( "input[name^='share']" ) Select all input elements that "name" attribute starts with "share"
f. Child Selector ("parent > child")
Selects all direct child elements specified by "child" of elements specified by "parent". Example:
$( "ul.nav > li" ) Select all list items that are children of <ul class="nav">
6. How to hide/show HTML element in jQuery?
Keyword:.hide(), .show(), .toggle()
Answer:
Use .hide(), .show(), .toggle() methods. For example:
$("showButton").click(function(){
$("div").show();
});
$("hideButton").click(function(){
$("div").hide();
});
$("toggleButton").click(function(){
$("div").toggle();
});
7. How to update HTML element text in jQuery?
Keyword:
.text(), .html()
Use .text() or .html() methods.
.text(): Get the combined text contents of each element in the set of matched elements, including their descendants, or set the text contents of the matched elements.
Example:
$("button1").click(function(){
$("#nav1").text("Question");
});
.html(): Get the HTML contents of the first element in the set of matched elements or set the HTML contents of every matched element.
Example:
$("button1").click(function(){
$("#nav1").text("<b>Keyword</b>");
});
8. What are the differences between .empty(), .remove() and .detach() methods in jQuery?
Answer:.empty(): Remove all child nodes of the set of matched elements from the DOM. This method removes not only child elements, but also any text within the set of matched elements.
.remove(): Similar to .empty(). This method removes the element itself, as well as everything inside it. In addition to the elements themselves, all bound events and jQuery data associated with the elements are removed.
.detach(): Similar to .remove(), except that .detach() keeps all jQuery data associated with the removed elements. This method is useful when removed elements are to be reinserted into the DOM at a later time.
9. How to make Ajax request in jQuery?
Keyword:
.ajax(),
.getJSON(), .load(), .get(), .post()
jQuery offers a couple of methods to make Ajax request:
.ajax(): Perform an asynchronous HTTP (Ajax) request. It is jQuery's low-level AJAX implementation. All jQuery AJAX methods use this method internally. This method is mostly used for requests where the other methods cannot be used.
$.ajax({
type: "POST",
url: "http://",
data: data,
success: function(){...},
error : function(){...},
complete : function(){...},
dataType: "json"
});
.getJSON(): Load JSON-encoded data from the server using a GET HTTP request.
.load(): Load data from the server and place the returned HTML into the matched element.
.get(): Load data from the server using a HTTP GET request.
.post(): Load data from the server using a HTTP POST request.
More JavaScript jQuery interview questions and answers: JavaScript Interview Notes
- What is the meaning of symbol $ in jQuery?
- How to use multiple jQuery version on the same page?
- What is CDN? Why use it?
- How to handle events in jQuery?
- What are the differences between .bind(), .live(), .delegate() and .on()?
- How to add HTML element in jQuery?
- How to check if an element is empty in jQuery?
- What are global Ajax event handlers in jQuery?
- ......
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.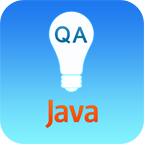
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
No comments:
Post a Comment