1. What is the difference between unbuffered I/O stream and buffered I/O stream?
Keyword:Unbuffered: read and write is handled directly by OS. InputStream, OutputStream.
Buffered: read and write on buffer. BufferedInputStream, BufferedOutputStream.
Unbuffered is less efficient than buffered.
Answer:
Unbuffered I/O means each read or write request is handled directly by the underlying OS. This can make a program much less efficient, since each such request often triggers disk access, network activity, or some other operation that is relatively expensive. Examples: InputStream, OutputStream, FileInputStream, FileOutputStream, Reader, Writer, FileReader, FileWriter.
To reduce this kind of overhead, the Java platform implements buffered I/O streams. Buffered input streams read data from a memory area known as a buffer; the native input API is called only when the buffer is empty. Similarly, buffered output streams write data to a buffer, and the native output API is called only when the buffer is full. To flush a stream manually, invoke its flush method.
Examples: BufferedInputStream, BufferedOutputStream, BufferedReader, BufferedWriter.
2. How to read and write text files using Java I/O?
Keyword:BufferedReader, readLine()
PrintWriter, println()
Answer:
Typically, we use buffered I/O streams to read/write text files.
Example:
import java.io.*;
public class TextFileRW {
public static void main(String[] args) {
BufferedReader br = null;
PrintWriter pw = null;
try {
br = new BufferedReader(new FileReader("a.txt"));
pw = new PrintWriter(new FileWriter("b.txt"));
String line;
while((line=br.readLine())!=null) {
pw.println(line);
}
}
catch(IOException e) {
e.printStackTrace();
}
finally {
if(br!=null) {
try{
br.close();
} catch(IOException e2) {
e2.printStackTrace();
}
}
if(pw!=null) pw.close();
}
}
}
3. How to read command line input in Java?
Keyword:a. InputStreamReader and system.in
b. Console class
c. Scanner class
Answer:
Java supports several ways to read command line input:
a. Access standard input through system.in, along with the InputStreamReader and BufferedReader classes.
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String input = null;
try {
input = br.readLine();
} catch (IOException e) { ... }
b. Using Console class.
Console console = System.console();
String input = console.readLine();
The Console is particularly useful for secure password entry:
char[] password = console.readPassword("Enter your password: ");
c. Using Scanner class.
Scanner sc = new Scanner(System.in);
int i = sc.nextInt();
String s = sc.next();
4. What is the difference between Stack and Heap memory in Java?
Keyword:stack: each thread has a private JVM stack, holds local variable, method invocation and return.
heap: shared among all thread, all class instances and arrays are allocated here.
Answer:
a. Each thread has a private JVM stack, created at the same time as the thread. A stack stores frames. It holds local variables and partial results, and plays a part in method invocation and return.
JVM has a heap that is shared among all threads. The heap is created on virtual machine start-up. The heap is the runtime data area from which memory for all class instances and arrays is allocated.
For example:
public class User {
int id; // instance variable
String name; // instance variable
public User(int id, String name) {
this.id = id;
this.name = name;
}
}
......
public static void main(String[] args) {
User user; // local variable - reference
user = new User(1, "Jerry");
int n = 2; // local variable - primitive
}
The reference variable "user" is stored on the stack, it refer to a User instance. The primitive variable "n" is stored on the stack.
The User instance itself (including instance variables "id" and "name") is stored on the heap.
b. Variables stored in stacks are only visible to the owner Thread, while objects created in heap are visible to all thread.
c. If there is no memory left in stack, JVM will throw java.lang.StackOverFlowError. If there is no more heap space for creating object, JVM will throw java.lang.OutOfMemoryError: Java Heap Space.
5. What is OutofMemoryError in Java? How to avoid it?
Answer:java.lang.OutOfMemoryError is a runtime error thrown when JVM cannot allocate an object because it is out of memory, and no more memory could be made available by the garbage collector.
Usually, there two types of java.lang.OutOfMemoryError:
OutOfMemoryError: Java heap space
OutOfMemoryError: PermGen space
If OutOfMemoryError: Java heap space occurs, you can solve it by increasing JVM heap size using JVM option: -Xmx
If OutOfMemoryError: PermGen space occurs, it means that you have exceeded Java's fixed 64MB block for loading class files, you can increase PermGen size using JVM option:
-XX:MaxPermSize=…
6. What is Garbage Collection?
Answer:Garbage collection (GC) is a form of automatic memory management. The garbage collector attempts to reclaim garbage, or memory occupied by objects that are no longer in use by the program.
In Java, GC is the process of looking at heap memory, identifying which objects are in use and which are not, and deleting the unused objects.
An in use object, or a referenced object, means that some part of your program still maintains a pointer to that object.
An unused object, or unreferenced object, is no longer referenced by any part of your program. So the memory used by an unreferenced object can be reclaimed.
7. Explain JVM options related to Garbage Collection.
Answer:-Xms256m or -XX:InitialHeapSize=256m
Set initial size of JVM heap (young + old).
-Xmx2g or -XX:MaxHeapSize=2g
Set max size of JVM heap (young + old).
-XX:NewSize=64m -XX:MaxNewSize=64m
Set absolute initial and max size of young generation (Eden + 2 Survivors).
-XX:NewRatio=2
Set ration of young vs old generation. NewRatio=3 means old generation will be twice the size of young generation.
-XX:SurvivorRatio=15
Set size of single survivor space relative to Eden space size.
-XX:PermSize=256m -XX:MaxPermSize=1g
Set initial and max size of permanent space.
8. What is the difference between Serializable and Externalizable interface?
Answer:Serializable interface is a marker interface, it does not contain any method.
Externalizable interface extends Serializable interface, adds two methods writeExternal() and readExternal() which are automatically called during serialization and deserialization.
Serializable interface provides a inbuilt serialization mechanism. Externalizable enables you to define custom serialization mechanism.
9. Describe different types of ClassLoader.
Keyword:Bootstrap ClassLoader
Extension ClassLoader
System ClassLoader
Answer:
There are three types of ClassLoader:
Bootstrap ClassLoader: load standard JDK class files from rt.jar and it is parent of all class loaders. Bootstrap class loader don't have any parents, if you call String.class.getClassLoader() it will return null.
Extension ClassLoader: load class form jre/lib/ext directory or any other directory pointed by java.ext.dirs system property.
System ClassLoader: load application specific classes from CLASSPATH environment variable, -classpath or -cp command line option, Class-Path attribute of Manifest file inside JAR.
10. How to create a custom ClassLoader?
Answer:First, extends java.lang.ClassLader or its subclass,
Then, set the parent class loader in the constructor,
Last, override the findClass() method to implement the custom load logic.
Example:
public class MyClassLoader extends ClassLoader {
public MyClassLoader() {
super(MyClassLoader.class.getClassLoader());
}
public Class findClass(String className) {
//custom load logic
//……
}
}
More Java IO, JVM & GC interview questions and answers: Java Interview Notes
- What is InputStream/OutputStream, Reader/Writer and InputStreamReader/OutputStreamWriter?
- How to copy files using Java I/O?
- What are transient variables? What role do they play in Serialization process?
- Explain the structure of Java heap.
- What is StackOverflowError? How to avoid it?
- When an object becomes eligible for garbage collection?
- What are WeakReference, SoftReference and PhantomReference?
- Explain Class Loader delegation model.
- How to explicitly load a class?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.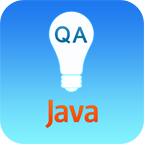
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play

No comments:
Post a Comment