1. Explain JSON data types.
Keyword:Number, String, Boolean, Array, Object, null
Answer:
JSON has the following basic data types:
a. Number — a signed decimal number that may contain a fractional part and may use exponential E notation.
b. String — a sequence of zero or more Unicode characters. Strings are delimited with double-quotation marks and support a backslash escaping syntax.
c. Boolean — either of the values true or false.
d. Array — an ordered list of zero or more values, each of which may be of any type. Arrays use square bracket notation with elements being comma-separated.
e. Object — an unordered associative array (name/value pairs). Objects are delimited with curly brackets and use commas to separate each pair, while within each pair the colon ':' character separates the key or name from its value.
f. null — An empty value, using the word null.
Example:
{
"id": 1,
"name": "iraylab",
"active": true,
"apps": [
{"name": "JavaScript Interview Notes", "version":"1.0"},
{"name": "Java Interview Notes", "version":"1.5"}
]
}
2. How to convert JSON text to JavaScript object?
Keyword:eval(),
JSON.parse()
Answer:
There are two ways to convert a JSON text into an object:
var myJSONtext = '{"id":1, "name":"JavaScript Interview Notes"}';
a. Use the eval() method:
var myObject = eval('(' + myJSONtext + ')');
b. Use JSON object and its parse() method:
try{
var myObject = JSON.parse(myJSONtext);
} catch(e){
console.log("Parse error:", e);
}
3. What is JSONP?
Answer:JSONP (JSON with padding) is a communication technique used in JavaScript programs running in web browsers to request data from a server in a different domain, something prohibited by typical web browsers because of the same-origin policy. JSONP takes advantage of the fact that browsers do not enforce the same-origin policy on <script> tags.
JSONP wraps up a JSON response into a JavaScript function and sends that back as a Script to the browser. A script is not subject to the Same Origin Policy and when loaded into the client, the function acts just like the JSON object that it contains.
4. How to parse an XML Document in JavaScript?
Keyword:Use browser built-in DOM parser
Answer:
All modern browsers have a built-in XML parser. Firefox, Chrome, and IE 9 support DOMParser object. For IE<9, we need to use loadXML() method.
For example:
var xmlStr = "<app><title>JavaScript Interview Notes</title><author>iraylab</author></app>";
var xmlDoc;
if (window.DOMParser) {
parser=new DOMParser();
xmlDoc=parser.parseFromString(txt,"application/xml");
}
else { // Internet Explorer < 9
xmlDoc=new ActiveXObject("Microsoft.XMLDOM");
xmlDoc.async=false;
xmlDoc.loadXML(txt);
}
5. How to manipulate XML Document in JavaScript?
Keyword:XMLDocument object
Answer:
XMLDocument object is a container object for an XML document, it provides a series of methods manipulate XML Document.
a. To create a new empty XMLDocument object, use the createDocument method;
b. To build an XMLDocument object from a string, use the DOMParser object. In IE<9, use loadXML method;
c. To build an XMLDocument object from a file or from a response of an HTTP request, use the XMLHttpRequest object and its responseXML property;
d. To get the root element of an XML document, use the documentElement property;
e. To retrieve an element in an XML document, use the firstChild, lastChild, nextSibling and previousSibling properties, the getElementsByTagName method and the childNodes collection.
6. What is Ajax?
Keyword:Asynchronous JavaScript and XML,
XMLHttpRequest,
XML, JSON
Answer:
Ajax is Asynchronous JavaScript and XML. Ajax is a group of interrelated Web development techniques used on the client-side to create asynchronous Web applications.
Ajax uses the XMLHttpRequest object to communicate with server-side scripts. It can make requests to the server and update portions of a page without reloading the whole page.
Ajax can send as well as receive information in a variety of formats, including JSON, XML, HTML, and even text files.
7. What is XMLHttpRequest?
Answer:XMLHttpRequest (XHR) is an API available to web browser scripting languages such as JavaScript. It is used to send HTTP or HTTPS requests to a web server and load the server response data back into the script. XMLHttpRequest is used heavily in AJAX programming.
In JavaScript, XMLHttpRequest is an object. XMLHttpRequest makes sending HTTP requests very easy. You simply create an instance of XMLHttpRequest, open a URL, send the request and handler the response when readyState changes.
8. How to make cross-browser Ajax request using XmlHttpRequest?
Keyword:new XMLHttpRequest()
new ActiveXObject("Microsoft.XMLHTTP") for IE
Answer:
Modern browsers (IE7+, Firefox, Chrome, Safari, and Opera) have a built-in XMLHttpRequest object, we can create XMLHttpRequest object directly. For old versions of Internet Explorer (IE5 and IE6), we need to create an ActiveX Object.
//Create cross-browser XmlHttpRequest instance:
var xhr;
if(window.XMLHttpRequest) { // Modern browsers, like IE8, firefox, etc
xhr = new XMLHttpRequest();
}
else if (window.ActiveXObject){ // for IE 6
xhr = new ActiveXObject("Microsoft.XMLHTTP");
}
if(xhr) {
//Register or define callback function:
xhr.onreadystatechange = function(){
if(xhr.readyState==4 && xhr.status==200) {
//handle response
}
}
//Send request:
xhr.open("GET", "http://www.iraylab.com/sample", true);
xhr.send();
}
More JavaScript JSON, XML & Ajax interview questions and answers: JavaScript Interview Notes
- What is JSON?
- Can you add comments to JSON?
- How to convert JavaScript object to JSON text?
- What are the difference between JSON and XML?
- List some technologies used by Ajax?
- What are the advantages and disadvantages of using Ajax?
- Explain XMLHttpRequest object common properties.
- Explain different type of ready state in XMLHttpRequest object.
- ......
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.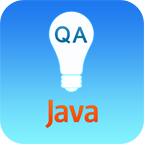
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
No comments:
Post a Comment