1. What is the relationship between JavaScript, JScript and ECMAScript?
Keyword:ECMAScript: language standard
JavaScript, JScript: different implementations
Answer:
ECMAScript is the name of the language standard developed by ECMA. The language is widely used for client-side scripting on the web, in the form of several well-known dialects such as JavaScript, JScript and ActionScript.
JavaScript is the Netscape/Mozilla implementation of the ECMA specification. JScript is the Microsoft implementation of the ECMAScript specification. While both JavaScript and JScript aim to be compatible with ECMAScript, they also provide additional features not described in the ECMA specifications.
2. What is the difference between undefined and null?
Keyword:undefined: declared,but no value; undefined type
null: a null value; object type
Answer:
a. undefined means a variable has been declared but has not yet been assigned a value. null is an assignment value. It can be assigned to a variable as a representation of no value.
b. For the data type, undefined is a type itself (undefined) while null is an object.
The following example shows their differences:
var a; //undefined variable
console.log(a); //output undefined
console.log(typeof a); //output undefined
var b = null;
console.log(b); //output null
console.log(typeof b); //output object
3. What is the difference between "===" and "=="?
Keyword:===: strict equality, no type conversion
==: try type conversion if not the same type. null == undefined
Answer:
The strict equality (===) returns true if the operands are strictly equal with no type conversion: If the two values have different types, they are not equal.
The equality operator (==) is similar to the strict equality operator, but it is less strict. If the values of the two operands are not the same type, it attempts some type conversions and then tries the comparison. If one value is null and the other is undefined, they are equal.
4. What is the difference between instanceof and typeof?
Keyword:instanceof: return true/fase,
typeof: return type string representation
Answer:
The instanceof operator returns true if the specified object is of the specified object type.
Usage: obj instanceof type
For example:
var today = new Date(2014, 12, 31);
if (today instanceof Date) {
//......
}
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned.
Usage: typeof operand //or: typeof (operand)
For example:
var str = "www.iraylab.com";
var num = 1;
var obj = {};
function hello() {
console.log("Hello world.");
}
typeof str; // returns "string"
typeof num; // returns "number"
typeof obj; // returns "object"
typeof hello; // returns "function"
typeof true; // returns "boolean"
typeof null; // returns “object"
5. How to convert a comma separated string from/to an array?
Keyword:split()
join()
Answer:
To convert a comma separated string to an array, use split() method:
var str = "a,b,c,d";
var arr = str.split(',');
To convert an array to a comma separated string, use join() method:
var arr = ["Apple", "Orange", "Banana"];
var str = arr.join(","); //the default separator is comma (,)
6. What is the difference between String literal and String object?
Keyword:String literal: string type
String object: object type
Answer:
String literals (denoted by double or single quotes) are primitive strings. JavaScript automatically converts primitives to String objects, so that it's possible to use String object methods for primitive strings. When a method is to be invoked on a primitive string or a property lookup occurs, JavaScript will automatically wrap the string primitive and call the method or perform the property lookup.
String literal is a type of string, while String object is a type of object:
var s1 = "iraylab.com";
var s2 = new String("iraylab.com");
console.log(typeof s1); // output "string"
console.log(typeof s2); // output "object"
7. How to create an array in JavaScript?
Keyword:array literal
new Array()
Answer:
There are two typical ways to create an array:
a. Using array literal:
var myArray1 = [1,2,5,6];
var myArray2 = ["Apple", "Orange", "Banana"];
b. Using new keyword:
var myArray3 = new Array(1,2,5,6);
var myArray4 = new Array("Apple", "Orange", "Banana");
8. How to add/remove elements from an array?
Keyword:push()
pop()
Answer:
Use push() and pop method:
var arr = ["Apple", "Orange", "Banana"];
//add element to the end of the array
arr.push("Pear");
//remove element from the end of the array
var element = arr.pop(); //return "Pear", arr is now ["Apple", "Orange", "Banana"]
9. What is associative array in JavaScript?
Keyword:object is associative array
Answer:
JavaScript doesn't have a specific associative array type, but every object has the ability to act as an associative array.
To create an associative array is to create a JavaScript object:
var obj ={};
obj["someKey"]=3;
obj["someOtherKey"]= someObject;
obj["anotherKey"]="Some text";
Access elements in the associative array:
var someValue = obj["someKey"];
obj["otherKey"]= someValue;
10. How to validate e-mail address using JavaScript?
Answer:Use regular expression and test() method. For example:
function validateEmail(email){
var reg = /^([A-Za-z0-9_\-\.])+\@([A-Za-z0-9_\-\.])+\.([A-Za-z]{2,4})$/;
if (!reg.test(email)) {
return false;
}
return true;
}
More JavaScript Basics interview questions and answers: JavaScript Interview Notes
- What is delete operator in JavaScript?
- What is || operator in JavaScript?
- How to generate a random integer value between two numbers?
- How to trim leading and trailing spaces from a string?
- What are the built-in types in JavaScript?
- What is the difference between slice(), substring() and substr()?
- How to sort an array of objects?
- How to create a Regular Expression in JavaScript?
- ......
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.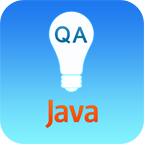
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
No comments:
Post a Comment