1. What is the difference between Classic Inheritance and Prototypical Inheritance?
Keyword:Classic Inheritance: class and object
Prototypical Inheritance: object, no class
Answer:
In Classic Inheritance, there are two types of abstraction: class and object. To create an object, first define the structure of the object, using a class declaration, then instantiate the class to create a new object. Objects created in this manner have their own copies of all instance attributes, plus a link to the single copy of each of the instance methods. Java and C# use classic inheritance.
In Prototypical Inheritance, there is only one type of abstraction: object. We create an object directly, instead of defining the structure through a class. The object can be reused by new objects. JavaScript use prototypical inheritance.
2. What are the different ways to create an object in JavaScript?
Keyword:object literal,
constructor function,
Object.create method
Answer:
There are three typical ways to create an object:
a. Using object literal
An object literal is a comma-separated list of colon-separated name:value pairs, enclosed within curly braces.
For example:
var myObject = {
property1 : "www.iraylab.com",
property2 : {
nestedProperty1 : "JavaScript"
}
};
b. Using constructor function
Define the object type by writing a constructor function. Then, create an instance of the object with new keyword.
For example:
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
var myCar = new Car("BMW", "X5", 2014);
c. Using the Object.create method
With ECMAScript 5, we can a static function Object.create() to create an object.
The Object.create() method creates a new object with the specified prototype object and properties: Object.create(prototype [, propertiesObject ])
For example:
var obj = Object.create(null, {
make: "BMW",
model: "X5",
year: 2014
});
3. How to access the properties of an object?
Keyword:dot notation .
bracket notation []
Answer:
There are two ways to access the properties of an object.
var car = {
make: "BMW",
year: 2014
};
a. Using the dot notation: .
var m = car.make; // get property value
car.make = "GM"; // set property new value
b. Using the bracket notation: []
var m = car["make"];
car["make"] = "GM";
4. How to define public and private properties/methods in an object?
Keyword:private: var
public: this
Answer:
In a JavaScript object, properties/methods defined with var keyword can only be accessed inside the object;
properties/methods defined with this keyword can be accessed from outside the object.
For example:
function Car (maker, model, year) {
//public properties and methods:
this.maker = maker;
this.model = model;
this.year = year;
this.getInfo = function() {
return "Car info: maker=" + this.maker + ", model="+ this.model +", year=" + this.year;
};
//private properties and methods:
var color;
var getColor = function() {
return "color=" + color;
};
//access a private method inside the object
this.getDetailedInfo = function() {
return this.getInfo() + ", " + getColor();
}
}
5. How to add custom methods and properties to an object?
Keyword:use prototype
Answer:
To add properties and methods to an object, we can modify its prototype property.
For example:
function Car (maker, model, year) {
this.maker = maker;
this.model = model;
this.year = year
}
//add new property and new method:
Car.prototype.color = "Black";
Car.prototype.getRating = function() {
return 1;
};
6. How to create a namespace in JavaScript?
Answer:In JavaScript, there is no the concept of namespace, we can create global object to emulate namespace.
For example, the following code create a namespace "iraylab.JSModule" and create two functions inside this namespace:
var iraylab = iraylab || {};
iraylab.JSModule = {
add: function(a, b) {
return a + b;
},
subtract: function(a, b) {
return a -b;
}
};
7. How to implement inheritance in JavaScript?
Keyword:use prototype and constructor function
Answer:
JavaScript is a class-free, object-oriented language, we can implement prototypal inheritance by using prototype and constructor function.
The following is an example to implement this kind of inheritance.
a. Define super class:
function Vehicle () {}
b. Define sub class:
function Car (maker, model, year) {
this.maker = maker;
this.model = model;
this.year = year
}
c. Set up inheritance:
Car.prototype = new Vehicle;
d. Extend super class using prototype:
Vehicle.prototype.getInfo = function(){
console.log("This is a vehicle.");
}
e. Sub class override super class's method:
Car.prototype.getInfo = function() {
console.log("This is a car.");
};
8. How to implement Singleton Pattern in JavaScript?
Answer:The singleton pattern is a design pattern that restricts the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system.
In JavaScript, we can implement a Singleton as follows:
var Singleton = (function () {
var instance;
function init() {
//define private methods and variables here...
function privateMethod(){
console.log( "a private method" );
}
var privateVariable = "a private variable";
return {
// Public methods and variables
publicMethod: function () {
console.log( "a public method" );
},
publicProperty: "a public property"
};
};
return {
// Get the Singleton instance if one exists or create one if it doesn't
getInstance: function () {
if ( !instance ) {
instance = init();
}
return instance;
}
};
})();
// Use the singleton class:
var mySingleton = Singleton.getInstance();
......
More Object-Oriented JavaScript interview questions and answers: JavaScript Interview Notes
- What is constructor in JavaScript?
- How to list all properties of an object itself?
- How to define static properties and methods in an object?
- What is the global object in JavaScript?
- What is prototype in JavaScript?
- What is the difference of using prototype and "this" to define a method?
- How to handle error in JavaScript?
- ......
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.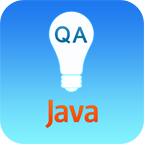
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
No comments:
Post a Comment