1. What is the difference between JVM, JRE and JDK?
Keyword:JVM: Execute Java bytecode
JRE = JVM + libraries
JDK = JRE + compiler + tools
Answer:
The Java Virtual Machine (JVM) is a virtual machine that can execute Java bytecode. It is the code execution component of the Java platform. The JVM is responsible for converting byte code into machine specific code, so we have different JVM for Windows and Linux platforms.
The Java Runtime Environment (JRE) provides the libraries, Java Virtual Machine (JVM), and other components to run Java programs. The JRE does not contain tools and utilities such as compilers or debuggers for developing Java programs. If you want to execute any Java program, the JRE should be installed.
The Java Development Kit (JDK) is a superset of the JRE, and contains everything that is in the JRE, plus tools such as the compilers and debuggers necessary for developing Java applications. If you want to write your own programs, and to compile them, the JDK should be installed.
2. Explain different access modifiers in Java
Keyword:public - all
private - within the class
protected - package + sub class
default - package
Answer:
There are 4 access modifiers to determine whether other classes can use a particular field or invoke a particular method:
public - public members are visible to all classes.
private - private members are visible only to its own class.
protected - protected members are visible to the classes within its own package and any subclasses.
default - default members are members that have no access modifier. They are visible only to the classes within its own package, not visible to subclasses.
3. What is the difference between final, finally and finalize?
Keyword:final - final variable, final method, final class
finally - exception handling
finalize - garbage collection
Answer:
The final keyword can be applied to variables, methods or classes.
The value of a final variable cannot be changed.
A final method cannot be overridden by other classes.
A final class cannot be inherited by other classes.
The finally block is used in exception handling. A finally block can be followed by try-catch or without catch block. It is guaranteed to be executed despite whether an exception is thrown or not. Putting cleanup code in a finally block is always a good practice, even when no exceptions are anticipated.
The finalize is a method used in garbage collection. It will be called by the garbage collector on an object when garbage collection determines that there are no more references to the object. A subclass overrides the finalize method to dispose of system resources or to perform other cleanup.
4. What is the difference between == and equals()?
Answer:The == operator compares two values to see if they refer to the same object.
The equals() method is defined in java.lang.Object class and it is used to check the equality of two objects. By default, the equals() method behaves the same as the "==" operator. But we usually override it to define equality based on object's business logic. For example:
java.lang.String overrides the equals() method to check if two strings represent the same sequence of characters.
5. What is the difference between checked exception and unchecked exception?
Answer:Checked exception includes the Exception class and any subclasses that are not subclasses of RuntimeException. Checked exceptions need to be declared in a method or constructor's throws clause if they can be thrown by the execution of the method or constructor. If an checked exception occurs, the program should catch the exception, either handle it or throw another exception that is either unchecked or declared in its throws clause.
Example: IOException, SQLException
Unchecked Exception is also called Runtime Exception. Unchecked exceptions do not need to be declared in a method or constructor's throws clause if they can be thrown by the execution of the method or constructor. If an unchecked exception occurs, the program doesn't have to handle it explicitly.
Example: NullPointerException, ArrayIndexOutofBoundException, UnsupportedOperationException.
6. Explain the basic concepts of OOP
Keyword:Encapsulation, Inheritance, Polymorphism, Abstraction
Answer:
Encapsulation is used to hide the values or state of a structured data object inside a class, preventing unauthorized parties' direct access to them. Publicly accessible methods are generally provided in the class (so-called getters and setters) to access the values, and other client classes call these methods to retrieve and modify the values within the object.
Inheritance is a kind of code reuse mechanism that allows to define a subclass of data object that share some or all of the characteristics of super class. Inheritance defines an is-a relationship between a superclass and its subclasses.
Polymorphism is the capability of different objects can respond to the same message in different ways, enable objects to interact with one another without knowing their exact type. Polymorphism in Java has two types: compile-time polymorphism (static binding) and run-time polymorphism (dynamic binding). Method overloading is an example of compile-time polymorphism, while method overriding is an example of run-time polymorphism.
Abstraction is the process of separating the essential characteristics of an object from the background details. Abstraction is used to reduce complexity and allow efficient design and implementation of complex software systems.
7. What is the relation between class and object?
Keyword:class - object template
object - class instance
Answer:
A class is a template for defining objects. It specifies the members and methods that can exist in an object. An object is an instance of a class. For example:
The Dog class, it could have members like color, age and methods like sit, bark.
Cody and Lucy could be two different objects of the Dog class, each one with different values for color and age. They're not the same at all, but they're both Dogs.
8. What is the difference between abstract class and interface?
Answer:a. Abstract class can have default implementations for some of its method, while interface can only have the signature of methods but no body.
b. Abstract class methods can have access modifiers as public, private, protected, static, but interface methods are implicitly public and abstract.
c. Abstract class can have constructors but interface cannot.
d. A class can implement multiple interfaces, but can extend only one abstract class.
9. What is the difference between method overloading and method overriding?
Answer:Method overloading means having two or more methods with the same name but with different signatures (different parameters list and different type of parameters). Method overloading is compile-time polymorphism.
Method overriding means having two methods with same name and same signature, one method in super class and the other in sub class. Method overriding is run-time polymorphism.
10. What is the difference between inheritance and composition?
Keyword:Inheritance: IS-A, compile-time
Composition: HAS-A, run-time
Answer:
a. Inheritance is used for "IS-A" relationship, while composition is used for "HAS-A" relationship.
Example:
class Vehicle {}
class Engine {}
class Car extends Vehicle {
private Engine engine;
}
Car is a kind of Vehicle, so we use inheritance to represent their relationship.
Car has an Engine, so we use composition to represent their relationship.
b. Inheritance is determined at compile time, while composition is determined at run time. With composition, it's easy to change the behavior on the fly with Dependency Injection.
More Java & OOP interview questions and answers: Java Interview Notes
- What is Java Platform?
- What is the difference between PATH and CLASSPATH?
- How are this() and super() used with constructors?
- What is static keyword?
- Explain Java Exception hierarchy
- What is the difference between throw and throws?
- Is the finally block guaranteed to be called?
- What are the benefits of OOP?
- What is the difference between Abstraction and Encapsulation?
- What is the difference between static binding and dynamic binding?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.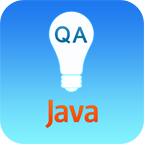
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play

No comments:
Post a Comment