1. Explain different ways of creating a thread.
Keyword:Thread class
Runnable interface
Answer:
a. extends Thread class:
public class MyThread extends Thread {
public void run(){
//thread execution logic
}
}
b. implements Runnable interface:
public class MyThread implements Runnable {
public void run(){
//thread execution logic
}
}
2. Explain the life cycle of thread.
Keyword:New, Runnable, Running, Blocked, Waiting, Dead
Answer:
There are the following states in the life cycle of thread:
New - This is the state when the thread instance has been created, but the start() method has not been invoked.
Runnable - This is the state when the thread is eligible to run, but the scheduler has not selected it to be the running thread. A thread enters the runnable state when the start() method is invoked. A thread can also return to the runnable state from a blocked, waiting, or sleeping state.
Running - This is the state when the thread scheduler selects it from the runnable pool to be the currently executing thread.
Blocked - This is the state when the thread is waiting for a monitor lock to enter a synchronized block/method or reenter a synchronized block/method after calling the wait() method.
Waiting - This is the state when the thread is waiting for another thread to perform a particular action. For example, a thread that has called Object.wait() on an object is waiting for another thread to call Object.notify() or Object.notifyAll() on that object. A thread that has called Thread.join() is waiting for a specified thread to terminate.
Dead - This is the state when the thread has completed execution.
3. What is the difference between sleep(), yield() and stop() methods?
Keyword:sleep: cause thread to stop executing, keep monitor.
yield: cause thread pause and allow other threads to execute, behavior not guaranteed.
stop: force thread to stop executing, unlock all monitors. deprecated.
Answer:
The sleep() method causes the current thread to stop executing for a given amount of time; if no other thread or process needs to be run, the CPU will be idle. The thread does not lose ownership of any monitors.
The yield() method hints the scheduler that the current thread is not doing anything particularly important and is willing to yield its current use of a processor. This method doesn’t guarantee that the current thread will pause. If there is no waiting thread or all the waiting threads have a lower priority, the current thread will continue its execution.
The stop() method forces the current thread to stop executing. Stopping a thread causes it to unlock all of the monitors that it has locked. This method is unsafe and has been deprecated.
4. What is the difference between start() and run() method?
Answer:The start() method causes the thread to begin execution and make the thread enter the runnable state. When the scheduler pickup the thread from the runnable pool, the run() method get executed.
The run() method is the thread's execution logic. Subclasses of Thread should override this method.
5. How to ensure that a thread runs after another thread?
Keyword:use join() method
Answer:
Use the join() method. This method wait for the thread on which it’s called to be dead or wait for a certain amount of time. It has three forms:
join() - waits for this thread to die.
join(long millis) - waits at most millis milliseconds for this thread to die. A timeout of 0 means to wait forever.
join(long millis, int nanos) - waits at most millis milliseconds plus nanos nanoseconds for this thread to die.
For the following example, t2 will start only when t1 is dead.
Thread t1 = new Thread();
Thread t2 = new Thread();
try {
t1.join();
} catch (InterruptedException e) {}
t2.start();
6. What is ThreadLocal? What is the difference with synchronized?
Keyword:thread-local variable, independent copy for each thread.
Servlet may use ThreadLocal to store local data.
ThreadLocal for data isolation, Synchronized for data share.
Answer:
ThreadLocal class provides thread-local variables. These variables differ from their normal counterparts in that each thread can access an independent copy of the variable. ThreadLocal instances are typically private static fields in classes that wish to associate state with a thread.
Example: a servlet may use ThreadLocal to store local data for each thread.
Main methods of ThreadLocal class:
get() - Returns the value in the current thread's copy of this thread-local variable.
set() - Sets the current thread's copy of this thread-local variable to the specified value.
initialValue() - Returns the current thread's "initial value" for this thread-local variable.
The difference with synchronized:
Synchronized is used to share data among threads, ThreadLocal is used to isolate data among threads.
7. What are DeadLock, LiveLock, and Starvation?
Answer:Deadlock describes a situation where two or more threads are blocked forever, waiting for each other to release lock.
LiveLook describes a situation where a thread acts in response to the action of another thread. If the other thread's action is also a response to the action of another thread, then livelock may result. The threads are not blocked, but they are too busy responding to each other to resume work.
Starvation describes a situation where a thread is unable to gain regular access to shared resources and is unable to make progress. This happens when shared resources are made unavailable for long periods by greedy threads.
8. How to solve the Producer-Consumer problem?
Keyword:a. Use wait(), notify()/notifyAll() methods.
b. Use BlockingQueue, put(), take().
Answer:
There are two typical ways to solve the Producer-Consumer problem:
a. Use wait and notify/notifyAll methods of Object to communicate between Producer thread and Consumer thread.
Sample code:
List<Element> queue = new LinkedList<Element>();
……
Producer thread:
while(true) {
synchronized(queue) {
while(queue.size() == MAX_SIZE) { //full
queue.wait();
}
queue.add(new Element(…)) //produce
}
}
……
Consumer thread:
while(true) {
synchronized(queue) {
while(queue.isEmpty()) { //empty
queue.wait();
}
Element e = queue.remove(0);
processElement(e); //consume
}
}
……
b. Use BlockingQueue and its blocking methods: put() and take()
Sample code:
BlockingQueue<Element> queue = new ArrayBlockingQueue<Element>();
……
Producer thread:
while(true) {
queue.put(new Element(…)) //produce
}
……
Consumer thread:
while(true) {
processElement(queue.take());
}
……
More Java Multithreading interview questions and answers: Java Interview Notes
- What is the difference between process and thread?
- What are the ways in which a thread can enter the waiting state?
- What is the difference between synchronized method and synchronized block?
- What is the difference between synchronized and volatile?
- Explain wait(), notify() and notifyAll() methods of Object class.
- What is the difference between Mutex and Semaphore?
- What is Lock in Java Concurrency API?
- What is ReentrantLock in Java Concurrency API?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.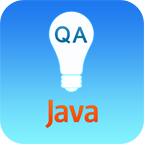
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play

No comments:
Post a Comment