1. What is the difference between creating String as new() and literal?
Keyword:string literal checks string pool first
new() create new object directly
Answer:
Creating String by a string literal, JVM checks the String Pool to see if an identical String already exists. If a match is found, the reference to the new literal is directed to the existing String, and no new String literal object is created.
While creating String with new(), JVM create a new String object instead of using an existing one from the String Pool.
2. Why always override hashCode() if overriding equals()?
Keyword:contract: equals() is true, hashCode() return same integer value.
default equals() and hashCode() implementations are based on memory address.
used as key in HashMap.
Answer:
One general contract of hashCode() is: if two objects are equal according to the equals(Object) method, then calling the hashCode method on each of the two objects must produce the same integer result.
The default implementation of equals() method just compares the memory addresses of the objects. And the default implementation of hashCode() method just returns an integer based on the identity of the object instead of instance variable values of the object. When the value of a instance variable changes, the hash code calculated by the default implementation does not change. This can have unexpected problems when the class is used as key in hash-based collections.
Example:
public class User {
private int id;
public User(int id) {
this.id = id;
}
public boolean equals(Object obj) {
if (!(obj instanceof User))
return false;
if (obj == this)
return true;
return this.id == ((User) obj).id;
}
public static void main(String[] args) {
Map<User, Object> map = new HashMap<User, Object>();
map.put(new User(123),"User Info");
String userInfo = map.get(new User(123)));
}
}
The value of userInfo is: null. This is because the hashCode() method is not overridden, the Object.hashCode returns two integer numbers that have no relation to the value of id, which violates the general contract of hashCode.
To fix the problem, you need to override the hashCode method like this:
public int hashCode(){
return this.id;
}
3. What is the difference between String, StringBuffer and StringBuilder?
Keyword:String: immutable
StringBuffer: mutable, synchronized
StringBuilder: mutable, non-synchronized
Answer:
String is immutable, if you try to change a string instance value, another object gets created.
StringBuffer and StringBuilder are mutable, so you can change their values.
StringBuffer is synchronized, while StringBuilder is not, which makes the performance of StringBuilder is better than StringBuffer.
4. How to define an immutable class?
Answer:a. Declare the class as final;
b. Make all fields final and private;
c. Set the value of fields use constructor only;
d. Don't provide any setter methods;
e. If the instance fields include references to mutable objects, don't allow those objects to be changed:
Don't provide methods that modify the mutable objects.
Don't share references to the mutable objects. Never store references to external, mutable objects passed to the constructor; if necessary, create copies, and store references to the copies. Similarly, create copies of your internal mutable objects when necessary to avoid returning the originals in your methods.
5. Describe the Java Collections Framework hierarchy.
Keyword:Collection:
List (ArrayList, LinkedList),
Set (HashSet, TreeSet),
Queue (PriorityQueue),
Deque (ArrayDeque)
Map: HashMap, TreeMap, LinkedHashMap
Java Collections Framework consists of a set of interfaces and implementations. Two root interfaces are: Collection and Map.
Collection interface has the following important sub interfaces and implementations:
List - A list is an ordered collection. Lists may contain duplicate elements. Two typical implementations are ArrayList and LinkedList.
Set - A Set is a Collection that cannot contain duplicate elements. Two typical implementations are HashSet and TreeSet.
Queue - A Queue is a collection for holding elements prior to processing. Queues typically order elements in a FIFO (first-in-first-out) manner. Two typical implementations are PriorityQueue and LinkedList.
Deque - A Deque is a double-ended-queue. A double-ended-queue is a linear collection of elements that supports the insertion and removal of elements at both end points. The Deque interface can be used both as last-in-first-out stacks and first-in-first-out queues. Two typical implementations are ArrayDeque and LinkedList.
Map interface maps keys and values. A map cannot contain duplicate keys; each key can map to at most one value. The Java platform contains three general-purpose Map implementations: HashMap, TreeMap, and LinkedHashMap.
6. How to remove duplicate elements from a List?
Answer:Use Set to remove duplicate elements.
For example:
List<String> list = new ArrayList<String>();
list.add("iraylab");
list.add("iraylab");
Set<String> set = new HashSet<String>();
set.addAll(list);
list.clear();
list.addAll(set);
7. How to convert an array from/to an ArrayList?
Keyword:Arrays.asList()
List.toArray()
Answer:
To convert an array to an ArrayList, use Arrays.asList method:
String[] arr = new String[]{"www", "iraylab", "com"};
ArrayList<String> list = new ArrayList<String>(Arrays.asList(arr));
To convert an ArrayList to an array, use toArray method of List:
List<String> list=new ArrayList<String>();
list.add("www");
list.add("iraylab");
list.add("com");
String[] arr = list.toArray(new String[list.size()]);
8. How to sort a list in reverse order?
Answer:Use java.util.Collections class.
This class consists exclusively of static methods that operate on collections: such as sort, search, reverse, shuffle, etc.
To sort a collection in reverse order, we invoke the reverse method:
List<String> list = new ArrayList();
list.add("abc");
list.add("cde");
list.add("efg");
Collections.reverse(list);
More Java Strings and Collections interview questions and answers: Java Interview Notes
- How do you check if two strings are equal?
- Why String is immutable in Java?
- What is the difference between List and Set?
- What is the difference between ArrayList and LinkedList?
- How HashMap works?
- How to create read only List, Set and Map?
- How to make a collection thread safe?
- What are the differences between HashSet, LinkedHashSet and TreeSet?
- ......
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.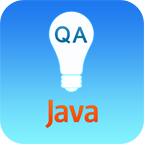
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play

No comments:
Post a Comment