1. How to add JavaScript to an HTML page?
Keyword:<script> tag,
external js file
Answer:
a. Use <script> tag in the HTML page:
In the following example, a JavaScript function is placed between the <script> and </script> tags. The function is invoked when a button is clicked.
<!DOCTYPE html>
<html>
<head>
<script>
function showHello() {
alert("hello");
}
</script>
</head>
<body>
<button type="button" onclick="showHello()">Show Hello</button>
</body>
</html>
b. Use external JavaScript file:
To use an external script, put the name of the script file in the source (src) attribute of the <script> tag.
<!DOCTYPE html>
<html>
<head>
<script src="hello.js"></script>
</head>
<body>
<button type="button" onclick="showHello()">Show Hello</button>
</body>
</html>
2. What is element, nodeList, attribute and namedNodeMap in HTML DOM?
Answer:Element refers to an HTML element in the DOM. Element objects can have child nodes of type element nodes, text nodes, or comment nodes. Element objects implement the DOM Element interface and also the more basic Node interface.
NodeList is an array of elements. Items in a nodeList are accessed by index in either of two ways: list.item(1) or list[1].
Attribute refers to an HTML attribute. An attribute belongs to an HTML element.
NamedNodeMap is an unordered collection of an elements attribute nodes. Items in a namedNodeMap are accessed by name or index.
3. How to find HTML element in an HTML page using DOM API?
Keyword:document.getElementById(),
Element.getElementsByTagName(),
document.getElementsByClassName()
Answer:
There are several ways to do this:
a. Find by id
Example: finds the element with id="add":
var e = document.getElementById("add");
b. Find by Tag Name
Example: finds the element with id="nav", and then finds all <p> elements inside "nav":
var navElement = document.getElementById("nav");
var elements = navElement.getElementsByTagName("p");
c. Find by Class Name
Example: find all HTML elements with class="category":
var elements = document.getElementsByClassName("category");
4. What is innerHTML?
Answer:The innerHTML is a property of HTML element object. It refers to the HTML code and the text that occurs between the element's opening and closing tag.
We can use innerHTML either to retrieve the current content of the element or to insert new content into that element.
The following example uses innerHTML to insert new elements to a HTML page:
<!DOCTYPE html>
<html>
<head>
<script>
function updateDiv(){
document.getElementById("div1").innerHTML = "<p>New title</p><p>New Text...</p>";
}
</script>
</head>
<body>
<div id="div1">Default Text</div>
<button type="button" onclick="updateDiv()">Update</button>
</body>
</html>
5. What is BOM?
Keyword:Browser Object Model,
window, navigator, location, document, history, screen, frames collection
Answer:
The Browser Object Model (BOM) is the part of JavaScript that allows JavaScript to interface and interact with the web browser. There are no official standards for the BOM.
At the top of the BOM is the window object. It represents the entire browser, with its toolbars, menus, status bar and the page itself. Under the window object, there are the following objects: navigator, location, document (DOM), history, screen, frames collection.
Using BOM, we can modify, move the window or can change the text in status bar, read the current URL, go back or forward of the current page which otherwise not possible with DOM.
6. What is the window object?
Keyword:window = web browser,
global variables/methods belong to window
Answer:
The window object represents the web browser itself. All global JavaScript objects, functions, and variables automatically become members of the window object. Global variables are properties of the window object. Global functions are methods of the window object. The document property points to the DOM document loaded in that window.
7. How to get web browser window size?
Keyword:use window object
Answer:
Use the window object innerWidth and innerHeight property.
var w = window.innerWidth; //return the width of browser window including the vertical scrollbar.
var h = window.innerHeight; //return the height of browser window including the horizontal scrollbar.
The innerWidth and innerHeight is not supported by in Internet Explorer < 9. To get the size of window in IE 6, 7, 8:
var w = document.documentElement.clientWidth;
var h = document.documentElement.clientHeight;
8. How to detect web browser information?
Keyword:use navigator object
Answer:
The navigator object contains information about the browser:
var browserName = navigator.appName; //returns the name of the browser.
var browserVersion = navigator.appVersion; //returns the version of the browser.
var userAgent = navigator.userAgent //returns the user agent string for the browser.
var cookieEnabled = navigator.cookieEnabled; //returns whether cookies are enabled in the browser.
9. What is DOM level 0 event handling model and DOM level 2 event handling model?
Keyword:DOM level 0: onclick="...", single event handler,
DOM level 2: addEventListener, removeEventListener, allow multiple event handlers
Answer:
DOM level 0 event handling model is based around the concept of using element attributes or named events on DOM elements. There are two model types: inline model and traditional model.
In inline model, event handlers are added as attributes of elements. For example:
<input type="button" onclick="handleButtonClickEvent();" />
In traditional model, event handlers can be added/removed by scripts. The event is added by assigning the handler name to the event property of the element object. For example:
<script>
document.onclick = function(){
//......
}
</script>
DOM level 2 event handling model defines an advanced event-handling API to manage events and subscriptions. With this model, we can add multiple event handlers for a single event.
There are three important methods:
addEventListener: Allows the registration of event listeners on the event target.
removeEventListener: Allows the removal of event listeners from the event target.
dispatchEvent: Allows sending the event to the subscribed event listeners.
10. How to add/remove JavaScript file to a web page at runtime?
Answer:Use DOM API. For example:
/* Add script to head element */
function addScript(loc) {
var script = document.createElement('script');
script.type = 'text/javascript';
script.src = loc;
document.getElementsByTagName('head')[0].appendChild(script);
return script;
}
/* Removes script from head element */
function removeScript(script) {
var head = document.getElementsByTagName('head')[0];
if (head && script) {
head.removeChild(script);
script = null;
}
}
/* Usage */
var myScript = addScript("http://www.iraylab.com/test/test.js");
......
removeScript(myScript);
More JavaScript DOM & BOM interview questions and answers: JavaScript Interview Notes
- What is the document object?
- How to change the style of an HTML element?
- How to change HTML content dynamically?
- How to detect the screen resolution?
- How to detect the operating system on the client machine?
- How to make web browser go back to previous page?
- What is DOM event?
- What is event bubbling and event capture?
- What are onload event and onunload event?
- ......
JavaScript Interview Notes
100+ frequently asked JavaScript interview questions with concise summaries and detailed answers. Topics include: JavaScript Basics, DOM, BOM, Object-Oriented JavaScript, Function, Scope, Closure, JSON, XML, Ajax, jQuery.Download on the AppStore Get it On Google Play
Java Interview Notes
300+ frequently asked Java interview questions with concise summaries and detailed answers. Topics include: Java & OOP, Strings & Collections, IO JVM & GC, Multithreading, Generics Reflection & Annotations, Design Patterns, Java EE, Spring, JPA & Hibernate.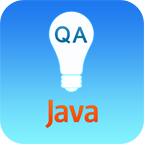
SQL Interview Notes
100+ frequently asked SQL and Database interview questions with concise summaries and detailed answers.Topics include: SQL Basic Concepts, SQL DDL & DML, Advanced SQL, Database Design and Performance Tuning.
Download on the AppStore Get it On Google Play
No comments:
Post a Comment